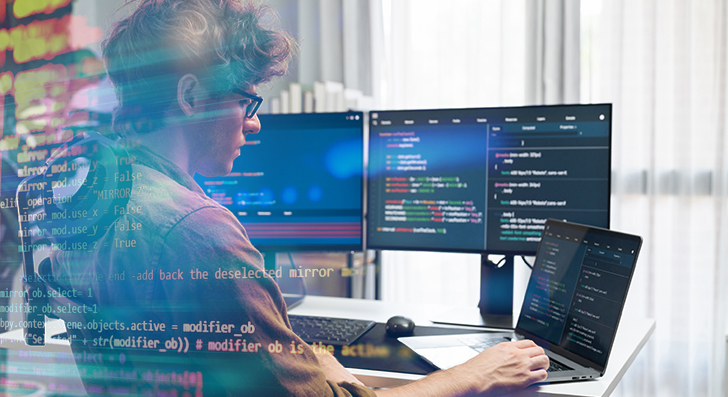
Scalability suggests your software can tackle expansion—far more customers, more details, plus more website traffic—with no breaking. As being a developer, setting up with scalability in mind saves time and pressure later. Right here’s a transparent and functional guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on afterwards—it should be aspect of one's approach from the beginning. Quite a few programs are unsuccessful once they grow rapid simply because the first style can’t cope with the extra load. Like a developer, you might want to Feel early regarding how your technique will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly connected. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial areas. Each module or support can scale on its own with out impacting The full procedure.
Also, consider your database from day just one. Will it need to handle a million buyers or just a hundred? Select the appropriate form—relational or NoSQL—dependant on how your data will expand. Program for sharding, indexing, and backups early, Even though you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only works under current conditions. Think of what would come about If the person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use layout designs that aid scaling, like message queues or party-pushed units. These assistance your application tackle more requests with out acquiring overloaded.
Any time you Establish with scalability in your mind, you are not just planning for achievement—you are decreasing long term headaches. A perfectly-prepared procedure is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal database is often a vital Component of constructing scalable programs. Not all databases are built a similar, and utilizing the Incorrect you can sluggish you down as well as trigger failures as your application grows.
Start off by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is an effective fit. These are typically robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle far more targeted traffic and data.
When your information is much more adaptable—like consumer exercise logs, item catalogs, or paperwork—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your browse and create designs. Are you presently executing lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Consider databases that will cope with high create throughput, or simply event-primarily based knowledge storage units like Apache Kafka (for temporary information streams).
It’s also wise to Assume in advance. You might not need Innovative scaling options now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data dependant upon your entry designs. And constantly watch databases effectiveness when you improve.
Briefly, the appropriate databases will depend on your app’s composition, velocity desires, And just how you be expecting it to improve. Just take time to choose properly—it’ll conserve lots of trouble later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s crucial to build economical logic from the beginning.
Commence by creating clean, uncomplicated code. Keep away from repeating logic and remove anything avoidable. Don’t select the most intricate Answer if a straightforward just one operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places where your code normally takes also extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be certain Each and every question only asks for the data you really need. Prevent Choose *, which fetches anything, and rather decide on specific fields. Use indexes to speed up lookups. And stay clear of undertaking a lot of joins, Specifically throughout large tables.
Should you see exactly the same knowledge being requested over and over, use caching. Retail store the outcomes quickly utilizing equipment like Redis or Memcached this means you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one by one, update them in teams. This cuts down on overhead and will make your application far more successful.
Make sure to test with big datasets. Code and queries that perform wonderful with a hundred documents could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly applications. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle more end users plus much more website traffic. If anything goes as a result of just one server, it will eventually immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As opposed to a single server performing all of the work, the load balancer routes buyers to unique servers based upon availability. This implies no single server receives overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts temporarily so it might be reused promptly. When consumers ask for the exact same details once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it through the cache.
There are two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your app extra economical.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does alter.
Briefly, load balancing and caching are simple but strong applications. With each other, they assist your application deal with far more customers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require tools that let your app increase quickly. That’s where cloud platforms and containers are available in. They provide you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When targeted traffic increases, you are able to include much more sources with only a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on setting up your app instead of running infrastructure.
Containers are A further critical Device. A container deals your app and all the things it ought to operate—code, libraries, settings—into one device. This causes it to be straightforward to move your application amongst environments, out of your notebook to the cloud, without the need of surprises. Docker is the most well-liked Device for this.
When your application makes use of numerous containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your application into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to mature devoid of limits, start off using these equipment early. They help you save time, minimize possibility, and help you keep centered on building, not repairing.
Watch Everything
Should you don’t observe your application, you won’t know when factors go read more Completely wrong. Monitoring aids the thing is how your app is executing, place challenges early, and make much better choices as your app grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—observe your application too. Keep an eye on how long it takes for customers to load webpages, how often mistakes occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes higher than a Restrict or maybe a company goes down, you'll want to get notified straight away. This allows you deal with difficulties rapidly, usually just before customers even notice.
Checking can be beneficial whenever you make modifications. For those who deploy a different attribute and see a spike in errors or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, traffic and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Ultimate Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off compact, Believe major, and build wise.